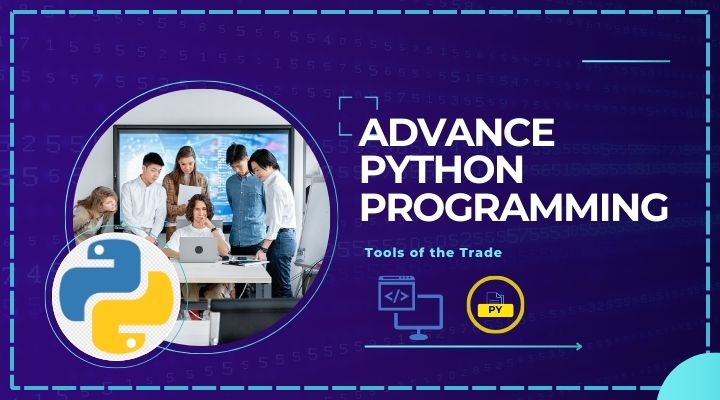
Advance Python Programming
Duration: 2 Months
Fee: 2500/- Per Month
This Python programming course is designed to provide a comprehensive introduction to Python, focusing on both theoretical concepts and practical applications. The course is structured to guide students from basic programming concepts.
Module 1: Introduction to Python
Lesson 1.1: What is Python?
- Overview of Python
- Python’s applications and industries
- Advantages of Python over other languages
- Installing Python and setting up the development environment (IDEs like VS Code, PyCharm, Jupyter Notebook)
Lesson 1.2: Python Syntax and Structure
- Python syntax basics (indentation and whitespace)
- Writing the first Python program (“Hello, World!”)
- Executing Python code (interactive mode vs script mode)
Module 2: Variables, Data Types, and Operators
Lesson 2.1: Variables and Data Types
- Variables in Python: definition, assignment, and rules
- Basic data types: integers, floats, booleans, and strings
- Type conversion (casting) between different data types
- Working with constants in Python
Lesson 2.2: Operators in Python
- Arithmetic operators (
+
,-
,*
,/
,//
,%
,**
) - Comparison operators (
==
,!=
,<
,>
,<=
,>=
) - Logical operators (
and
,or
,not
) - Bitwise operators (
&
,|
,^
,<<
,>>
) - Membership operators (
in
,not in
) - Identity operators (
is
,is not
)
- Arithmetic operators (
Module 3: Control Flow and Loops
Lesson 3.1: Conditional Statements
- The
if
,elif
, andelse
statements - Nested conditions
- The
pass
keyword and short-circuiting in logical operations
- The
Lesson 3.2: Looping Structures
for
loop (iterating over sequences)while
loop (executing based on conditions)- Loop control:
break
,continue
, andelse
in loops - Nested loops and iterating through lists, tuples, and dictionaries
Module 4: Functions
Lesson 4.1: Introduction to Functions
- Defining functions using the
def
keyword - Parameters and return values
- Default, keyword, and variable-length arguments
- Lambda functions (anonymous functions)
- Defining functions using the
Lesson 4.2: Scope and Lifetime of Variables
- Local and global variables
- The
global
keyword - Function scope, argument passing, and mutability
Lesson 4.3: Recursion
- Understanding recursion
- Base case and recursive case
- Recursive function examples (factorial, Fibonacci sequence)
Module 5: Data Structures
Lesson 5.1: Lists
- Defining and manipulating lists
- List operations:
append()
,extend()
,remove()
,pop()
, etc. - List slicing and indexing
- Nested lists and list comprehensions
Lesson 5.2: Tuples
- Introduction to tuples (immutable sequences)
- Accessing and manipulating tuples
- Tuple packing and unpacking
Lesson 5.3: Dictionaries
- Defining and accessing dictionaries
- Dictionary methods:
get()
,pop()
,keys()
,values()
, etc. - Iterating over dictionary keys and values
- Nested dictionaries
Lesson 5.4: Sets
- Defining and manipulating sets (unordered collections)
- Set operations (union, intersection, difference, etc.)
- Set methods:
add()
,remove()
,discard()
,clear()
Module 6: String Manipulation
Lesson 6.1: String Basics
- Creating and accessing strings
- String concatenation and repetition
- String indexing and slicing
- Escape sequences and special characters
Lesson 6.2: String Methods
- Common string methods:
lower()
,upper()
,split()
,replace()
,join()
, etc. - String formatting techniques (f-strings,
format()
,%
formatting)
- Common string methods:
Lesson 6.3: Regular Expressions (Optional)
- Introduction to regular expressions
- Using the
re
module - Basic patterns and searching, matching, and replacing
Module 7: File I/O (Input/Output)
Lesson 7.1: Reading Files
- Opening and reading files using
open()
- Reading content using
read()
,readline()
, andreadlines()
- Working with different file types (text, CSV)
- Opening and reading files using
Lesson 7.2: Writing Files
- Writing to files using
write()
andwritelines()
- Appending to files
- The
with
statement for automatic file closing
- Writing to files using
Lesson 7.3: File Handling Techniques
- Checking if a file exists with
os.path
- File and directory operations using the
os
module - Working with CSV files using the
csv
module
- Checking if a file exists with
Module 8: Error Handling and Exceptions
Lesson 8.1: Introduction to Exceptions
- What are exceptions?
- Built-in exceptions in Python
- Handling exceptions with
try
,except
,else
, andfinally
blocks
Lesson 8.2: Raising Exceptions
- The
raise
keyword and custom exceptions - Using
assert
statements for debugging
- The
Lesson 8.3: Exception Hierarchy
- Exception class hierarchy and handling multiple exceptions
- Creating custom exception classes