Blog
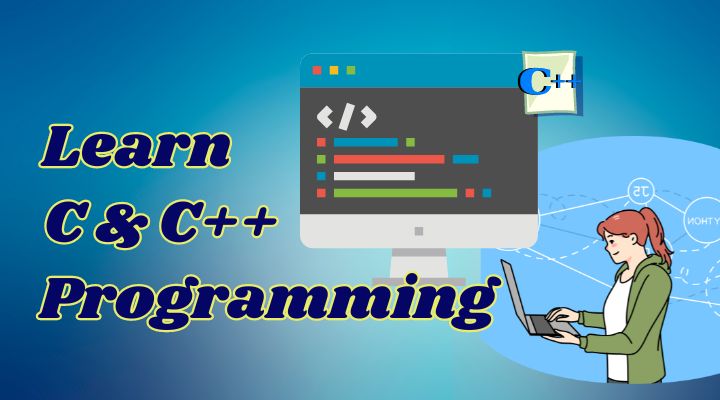
C & C++ Programming
Duration: 2 Months
Fee: 1000/- Per Month
Overview
Curriculum
Overview
This course is designed to provide comprehensive knowledge of C and C++ programming languages, from basic syntax and concepts to advanced topics such as data structures, algorithms, object-oriented programming (OOP), and memory management.
Curriculum
Module 1: Introduction to Programming in C
Basics of C Language
- Overview of C Programming
- History and Importance of C
- Setting up the Environment
- Structure of a C Program
- Compiling and Running C Programs
Data Types and Variables
- Basic Data Types (int, float, char, etc.)
- Declaring and Initializing Variables
- Constants and Literals
- Input and Output in C (scanf and printf)
Operators and Expressions
- Arithmetic Operators
- Relational and Logical Operators
- Increment/Decrement Operators
- Bitwise Operators
Control Flow Statements
- Conditional Statements: if, else, switch
- Loops: for, while, do-while
- Nested Loops
- Break, Continue, and Goto Statements
Functions in C
- Function Declaration and Definition
- Function Arguments and Return Values
- Passing Arguments by Value and Reference
Arrays and Strings
- Declaring and Initializing Arrays
- Accessing Array Elements
- Multi-dimensional Arrays
- Strings and String Handling Functions
Pointers
- Introduction to Pointers
- Pointer Arithmetic
- Pointers and Arrays
- Pointers and Functions
File Handling in C
- Opening and Closing Files
- Reading from and Writing to Files
- File Pointers
- Error Handling in File Operations
Module 2: Programming with C++
Introduction to C++
- Overview of C++ and Evolution from C
- Key Features of C++
- Structure of a C++ Program
- Input/Output in C++ (cin, cout)
Object-Oriented Programming (OOP)
- Introduction to OOP Concepts
- Classes and Objects
- Data Members and Member Functions
- Access Specifiers (Public, Private, Protected)
- Constructors and Destructors
Inheritance
- Types of Inheritance (Single, Multiple, Multilevel, Hierarchical, Hybrid)
- Base and Derived Classes
- Function Overriding
- Constructors in Derived Classes
Polymorphism
- Compile-Time Polymorphism (Function Overloading, Operator Overloading)
- Runtime Polymorphism (Virtual Functions, Abstract Classes)
- Pointers to Objects
- this Pointer
Encapsulation and Abstraction
- Concept of Encapsulation
- Data Hiding and Access Control
- Abstract Classes and Interfaces
ahirwardevendra12
0